Welcome to my in-depth analysis of the lexical environment in JavaScript!
As a developer, comprehending the concept of lexical environment is essential for creating optimized and effective code. This blog post will examine everything you need to know about a lexical environment, its operations, and its use in different language concepts.
By the end of this post, I hope you’ll have a solid understanding of lexical environments. So, let’s dive in!
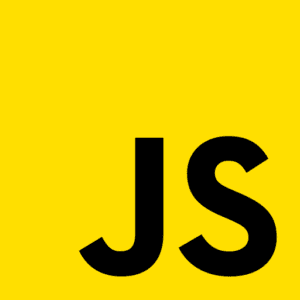
Lexical Environment
In JavaScript, a lexical environment is a data structure that stores all variables and function declarations. It allows the interpreter to recognize which variables or functions are accessible in different scopes within your program.
A lexical environment consists of two components: an environment record and a reference to the outer environment.
- An environment record is an object that stores the variables and functions that are declared within the current lexical environment.
- The reference to the outer environment is a pointer to the parent lexical environment, allowing the interpreter to access variables and functions defined in parent scopes.
A new lexical environment is formed every time a function or block is executed. The environment record of this lexical environment will contain all the variables and functions declared within that particular function or block. For example, when the following code is executed:
function lexicalFn() { let a = 1; let b = 3; }
A new lexical environment is constructed for the function lexicalFn()
. The environment record of this lexical environment will contain two properties: a
with a value of 1
and b
with a value of 3
.
When a variable or function is requested, the interpreter searches in the current lexical environment. If not found there, it proceeds to look through each parent lexical environment until the variable or function is located or reaches the global scope with no results. Lexical environments give you a way to determine what variables and functions are accessible as well as their scopes within your programs.
Execution Context
JavaScript’s execution context is an abstract concept that stands for the environment in which code is run. It’s how the interpreter tracks the program’s progress as it’s being executed. Every time a function or block is run, a new execution context is formed.
For each execution context, a related lexical environment supplies the variables and functions that can be utilized within the context. Whenever a function is called, a new execution context and a fresh lexical environment are generated. The environment record of the new lexical environment is populated with the variables and functions declared within the function.
As the interpreter executes the code, it uses the lexical environment to determine the scope of variables and functions and resolve references to variables and functions declared in parent scopes.
Scope
In JavaScript, the visibility of variables and functions is determined by the scope, which affects its accessibility in different parts of a program. The lexical environment captures where variables and functions are declared to establish their respective scopes. When a variable or function is declared in a particular lexical environment, it is said to be in the scope of that environment. A variable or function in the scope of a specific lexical environment is accessible within that environment and any nested environments.
A variable or function declared in a global lexical environment can be referred to as being in the global scope, and its access extends over every part of the program. In contrast, when something is declared within a function lexical environment, it’s said to have been placed in the function scope – limiting its accessibility only to this particular area and any other nested environments.
For example, when the following code is executed:
var globalVar = 'global'; function exampleFn() { var localVar = 'local'; }
The variable globalVar
is in the global scope and is accessible from any lexical environment in the program. The variable localVar
is in the local scope and is only accessible within the lexical environment of the exampleFn
function.
From ES6, JavaScript also introduced the concept of block scope, which means that variables declared with let
or const
within a block {}
are not accessible outside that block, unlike var
, which is function-scoped. For example, when the following code is executed:
var globalVar = 'global'; function exampleFn() { if (true) { let blockVar = 'block'; } console.log(blockVar); } exampleFn() // ReferenceError: blockVar is not defined
The variable blockVar
is in the block scope and is only accessible within the block in which it is declared. Attempting to access it outside of that block will result in a ReferenceError
.
Closures
In JavaScript, a closure is a feature that allows a function to not only access variables and functions within its own lexical scope, but also to maintain that access even if the function is called outside of its original environment. A closure is created by defining a function inside of another function.
A closure holds on to its connection to its lexical environment, allowing it to access the variables and functions that existed at its creation. Consequently, closures are capable of “retaining” their condition, even when they are called in a different situation.
For instance, consider the following code:
function outerFn() { let x = 7; return function innerFn() { console.log(x); }; } let closure = outerFn(); let x = 9; closure(); // 7
In this example, the inner function innerFn
is defined inside the outer function outerFn
. When outerFn
is invoked, it creates a new lexical environment and declares a variable x
with a value of 7
. The inner function innerFn
is returned and assigned to the variable closure
.
When the closure
function is invoked later, it still has access to the variable x
in the lexical environment created when outerFn
was invoked. This is because the inner function innerFn
has a closure over that lexical environment.
Hoisting
In JavaScript, hoisting refers to the process of moving variable and function declarations to the top of their scope, regardless of their original position in the code. It means you can use variables and functions before they are declared in the code.
Variables declared using the var
keyword are lifted to the beginning of their scope. This means they are accessible throughout their scope, even before they are declared. For example, in the following code:
console.log(y); // undefined var y = 20;
The variable y
is hoisted to the top of the scope, meaning it is accessible before it is declared. However, its value is undefined
until it is assigned later.
On the other hand, variables declared with let
and const
are not hoisted to the top of the scope. This means that they are not accessible before they are declared, and trying to access them before the declaration raises a ReferenceError
.
console.log(y); // ReferenceError: y is not defined let y = 20;
Function declarations are also hoisted to the top of their scope, so they can be invoked before they are defined in the code. For example, in the following code:
hoistedFn(); // "This is a hoisted function" function hoistedFn() { console.log('This is a hoisted function'); }
The function hoistedFn
is hoisted to the top of the scope and can be invoked before it is defined.
Hoisting is a critical concept to understand in JavaScript because it affects the behavior of variables and functions and can lead to unexpected results if not taken into account.
this
Keyword
The this
keyword in JavaScript is a reference to the current execution context. It is used to access the properties and methods of the object that the function is a method of or the object that the function is called on.
The value of the this
keyword is determined by the way the function is called. In most cases, the value of this
is determined by the object that the function is a method of or the object that the function is called on.
For example, when the following code is executed:
let obj = { a: 2, b: 4, getSum: function () { return this.a + this.b; }, }; console.log(obj.getSum()); // 6
The this
keyword inside the getSum
function refers to the obj
object, which allows the function to access the a
and b
properties of the object.
In cases where a function is not a method of an object, the value of this is determined by the object that the function is called on. For instance, in the following code:
let a = 2; let b = 4; let getSum = function () { return this.a + this.b; }; console.log(getSum.call({ a: 2, b: 4 })); // 6
The call
method allows you to call a function with a specific this
value. In this case, the function getSum
is called with the this
value of the object {a:2, b:4}
which allows the function to access the a
and b
properties of the object.
The this
keyword is a powerful and important feature of JavaScript, but it can also be one of the most confusing. Understanding how the this
keyword works and how it is affected by lexical environments is crucial for writing efficient and effective JavaScript code.
Strict Mode
Strict mode is not directly connected to a lexical environment, but it can have an influence on how the code is executed within that environment.
To enable strict mode, you can include the string 'use strict'
at the top of a script or function. For example:
'use strict'; function strictModeFn() { // code in strict mode } // or function strictModeFn() { 'use strict'; // code in strict mode }
Strict mode offers an upgrade for how certain parts of the language function, making it more reliable and effective. Certain risks and issues with JavaScript are removed when strict mode is enabled, and previous silent behaviors in non-strict mode will now throw errors.
For instance, when a variable is accessed in a lexical environment, and it’s not present, the interpreter will look in the parent lexical environment and keep searching through the sequence of parent environments until the variable is located or the global environment is reached. However, if strict mode is enabled, it will raise a ReferenceError
if the variable cannot be found.
Moreover, strict mode modifies how the this
keyword behaves in a lexical environment and makes it more predictable.
Using strict mode is not mandatory; however, you should take advantage of its security and efficiency benefits.
Lexical Environment in JavaScript: Conclusion
In this blog post, we delved into the intricacies of lexical environments in JavaScript, a fundamental concept that plays a critical role in determining the accessibility and behavior of variables, functions, and other identifiers in a program. We explored the mechanisms by which they are created and maintained, and how they are utilized in different parts of the language.
Here are some online resources to learn more about the lexical environment in JavaScript:
- You Don’t Know JS: Scope & Closures
- Understanding JavaScript Execution Context and Lexical Environment
Thank you for reading! I welcome any questions or comments. With a deeper understanding of lexical environments, you’ll be well on your way to writing more efficient, effective, and secure JavaScript code.