Have you ever encountered a tricky bug in your NodeJS application, only to find that you don’t have enough information to diagnose and fix the issue? This is where logging comes in. Logging is the process of recording messages during runtime to help developers identify problems in their applications. By adding logging to your application, you can gain insight into what is happening during runtime and better diagnose any issues that may arise.
NodeJS logging to files is made easy with built-in modules like fs
and popular third-party libraries, such as Winston or Pino. Logging can be used to capture various types of events, such as HTTP requests, database queries, and application errors. By logging these events, you can better understand how your application is behaving and identify issues that need to be addressed.
One of the most useful logging techniques is logging into a file. This allows you to store logs in a separate file, which can be helpful in debugging and monitoring your application. In this blog post, we’ll explore how to implement logging to files in NodeJS, both with the built-in modules and the popular logging library Winston. After reading this post, you’ll be equipped with the knowledge and tools you need to implement logging in your own NodeJS projects.
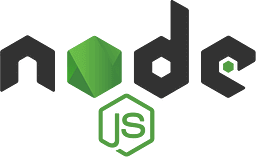
Logging to a File in NodeJS
Simple Logging with Built-in Modules
The simplest way to log to a file in NodeJS is to use the built-in fs
module to create a write stream and write messages to it. Here’s an example function that logs a message to a file named logs.txt
:
const fs = require('fs'); function logToFile(message) { const logStream = fs.createWriteStream('logs.txt', { flags: 'a' }); logStream.write(`${message}\n`); logStream.end(); } logToFile('Application started');
Content of the logs.txt
file:
Application started
This code creates a new write stream to the file logs.txt
with the a
flag, which appends data to the file if it already exists, or creates it if it doesn’t. The logToFile
function writes the message
argument to the log file, followed by a newline character, and closes the stream.
Logging with Multiple Levels
In more complex applications, it’s often beneficial to log messages with different levels of severity. For example, you might want to log informational messages, warnings, and errors separately to make it easier to identify and troubleshoot issues.
Here’s an example of a logger object that supports different levels of logging:
const fs = require('fs'); function logToFile(message) { const logStream = fs.createWriteStream('logs.txt', { flags: 'a' }); logStream.write(`${message}\n`); logStream.end(); } const logger = { info: (message) => logToFile(`[INFO] ${message}`), warn: (message) => logToFile(`[WARN] ${message}`), error: (message) => logToFile(`[ERROR] ${message}`), }; logger.info('Application started'); logger.warn('This could be risky'); logger.error('Something went wrong');
Content of the logs.txt
file:
[INFO] Application started [WARN] This could be risky [ERROR] Something went wrong
This code defines a logger
object that has methods for logging messages with different levels of severity. Each method calls the logToFile
function with a message that includes the level in brackets.
Extracting the Logger to a Separate File
Defining the logger in a separate file is useful, allowing it to be reused across different modules. The following example demonstrates a module that exports a logger
object:
const fs = require('fs'); function logToFile(message) { const logStream = fs.createWriteStream('logs.txt', { flags: 'a' }); logStream.write(`${message}\n`); logStream.end(); } const logger = { info: (message) => logToFile(`[INFO] ${message}`), warn: (message) => logToFile(`[WARN] ${message}`), error: (message) => logToFile(`[ERROR] ${message}`), }; module.exports = logger;
To use this logger in another module, you can require the logger.js
file and call its methods:
const logger = require('./logger'); logger.info('Application started'); logger.warn('This could be risky'); logger.error('Something went wrong');
With this setup, you can easily change the logging behavior of your application by editing the logger.js
file without changing the code in your application’s other modules. For instance, you can update logger.js
to write info, warnings, and error messages to different files.
Using a dedicated logging library can provide even more features and flexibility, such as logging to multiple files or transports, adding metadata to log messages, and using custom log formats. In the next section, we will explore how to use Winston to implement logging to a file in NodeJS.
Logging to a File with Winston
Winston is a popular logging library for NodeJS that provides many advanced logging features, including logging to a file. Winston can be easily configured to write logs to a file, making it an excellent choice for implementing logging in your NodeJS application. Here’s how you can set up logging to a file with Winston:
Install Winston as a dependency in your NodeJS project using the npm package manager:
npm install winston // or yarn add winston
Here’s an example of how to use Winston to log messages to a file:
const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), defaultMeta: { service: 'payment-service' }, transports: [ // Write all logs with importance level of `error` or less to `error.log` new winston.transports.File({ filename: 'error.log', level: 'error' }), // Write all logs with importance level of `info` or less to `combined.log` new winston.transports.File({ filename: 'combined.log' }), ], }); logger.info('This is an informational message'); logger.warn('This is a warning message'); logger.error('This is an error message');
Content inside error.log
:
{"level":"error","message":"This is an error message","service":"payment-service"}
Content of combined.log
:
{"level":"info","message":"This is an informational message","service":"payment-service"} {"level":"warn","message":"This is a warning message","service":"payment-service"} {"level":"error","message":"This is an error message","service":"payment-service"}
This code creates a new logger object with the winston.createLogger
method, which takes an options object that configures the logger. The level
option specifies the minimum level of logs to write, and the transports
option is an array of transports that define where logs are written. In this example, logs with a level of error
or less are written to error.log
, and logs with a level of info
or less are written to combined.log
. The format
option defines the format of the log messages, and the defaultMeta
option specifies default metadata to include with each log message.
The logger object has methods for logging messages at different levels, including logger.info
, logger.warn
, and logger.error
. When you call one of these methods with a message string, Winston formats the message and writes it to the appropriate transport(s).
With Winston, you can easily configure your loggers to write to multiple destinations, including files, console, and third-party logging services. You can also define custom log levels, filters, and formatters to suit your specific logging needs.
Debugging with Logs
Logs can be an invaluable tool for debugging NodeJS applications. By logging important information and events throughout the application’s execution, you can gain insights into how the application is functioning and identify issues that need to be addressed. Here are a few examples of how logs can be used for debugging:
- Tracing the flow of execution: By logging the start and end of functions and important code blocks, you can get a better understanding of how the application is executing and trace the flow of control through the code. This can help you identify issues such as functions that are not being called or code blocks that are executing in an unexpected order.
- Capturing errors and exceptions: By logging error messages and stack traces when exceptions are thrown, you can gain insights into what went wrong and where. This can help you identify and fix bugs in your code more quickly and effectively.
- Monitoring application state: By logging important metrics and state information, such as the contents of important variables or the number of active connections to a database, you can get a better understanding of how your application is functioning and identify potential issues before they become critical.
When using logs for debugging, there are a few best practices to keep in mind:
- Use meaningful log messages: Your log messages should be descriptive and informative, making it easy to understand what’s happening in your application just by reading the logs.
- Log relevant information: Don’t clutter your logs with irrelevant details that won’t be helpful for debugging. Instead, focus on logging the most important events and data that will help you diagnose issues in your application.
- Use appropriate log levels: Use different log levels (e.g. “info”, “warn”, “error”) to indicate the severity of the logged event. This can help you quickly identify the most critical issues in your logs.
- Avoid logging sensitive information: Make sure to avoid logging sensitive data, such as passwords or user data, which could be a security risk if the logs are accessed by unauthorized individuals.
By following these best practices and using logs effectively, you can make the debugging process much easier and more efficient, helping you to identify and fix issues in your NodeJS application more quickly and effectively.
NodeJS Logging to File: Recap
In this article, we’ve explored the importance of logging for debugging NodeJS applications and provided step-by-step guides to implement logging to a file both with built-in modules and the popular Winston library. By logging important information and events in your application, you can gain insights into how it’s functioning and identify and fix issues more quickly and effectively.
Logging to a file is a powerful technique for debugging and can be implemented with relative ease in NodeJS. By choosing the right approach and following best practices for logging, you can make your debugging process more efficient and effective.
Remember to use meaningful log messages, log only relevant information, use appropriate log levels, and avoid logging sensitive data. With these best practices in mind, you can use logs to trace the flow of execution, capture errors and exceptions, and monitor the application state to identify and fix bugs in your NodeJS application more quickly and effectively.
I hope this guide has been helpful in showing you how to implement logging to a file in NodeJS, and that you feel more confident in using logs to debug your applications.
If you want to learn more about NodeJS development, check out our blog post on the top 10 Node JS API Frameworks in 2023. Happy coding!